Forms are really important in any website for login, signup, or whatever. It is easy to make a form in HTML but forms in React work a little differently. In HTML the form data is usually handled by the DOM itself but in the case of react the form data is handled by the react components. All the form data is stored in the react’s component state, so it can handle the form submission and retrieve data that the user entered. To do this we use controlled components.
React uses the form to interact with the user and provides additional functionality such as preventing the default behavior of the form which refreshes the browser after the form is submitted.
Controlled Components: In simple HTML elements like input tags, the value of the input field is changed whenever the user type. But, In React, whatever the value the user types we save it in state and pass the same value to the input tag as its value, so here DOM does not change its value, it is controlled by react state. If you want to learn more about controlled components you can check the article Controlled Components in ReactJS. This may sound complicated But let’s understand with an example.
Example 1: In this example code, we are going to console log the value of the input field with the help of the onInputChange function. and we’ll see that every time the user input we get the output on the console of the browser.
First, create react app, and from your project directory update your index.js file from the src folder.
src index.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; class App extends React.Component { onInputChange(event) { console.log(event.target.value); } render() { return ( <div> <form> <label>Enter text</label> <input type="text" onChange={this.onInputChange}/> </form> </div> ); } } ReactDOM.render(<App />, document.querySelector('#root')); |
Output:
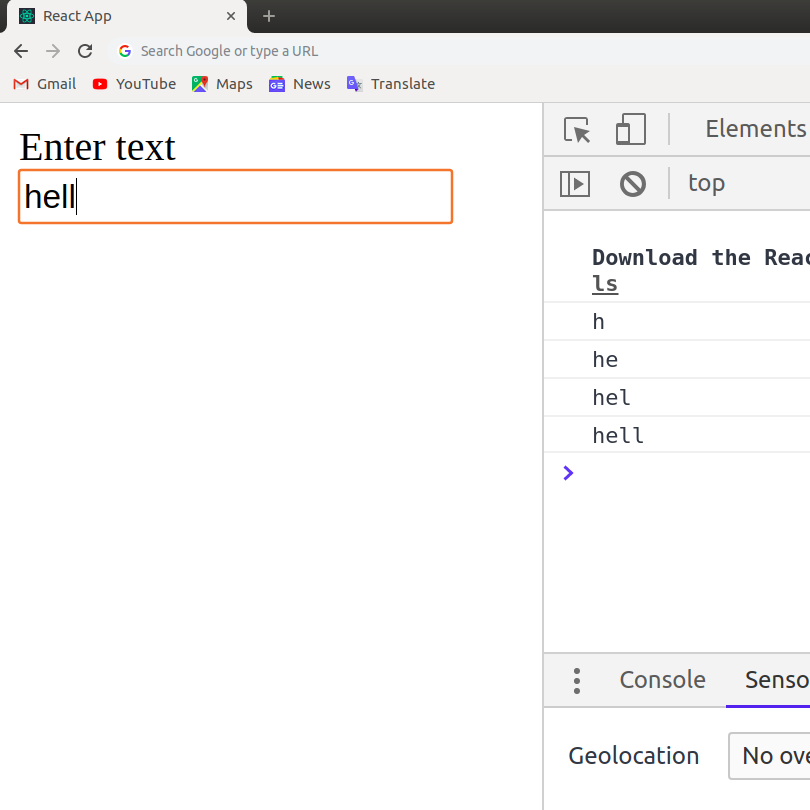
Example 2: Now let’s, again change the src/index.js with the below-given code, and in this code, we are updating the value of inputValue each time user changes the value in the input field by calling the setState() function.
Src index.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; class App extends React.Component { state = { inputValue: '' }; render() { return ( <div> <form> <label> Enter text </label> <input type="text" value={this.state.inputValue} onChange={(e) => this.setState( { inputValue: e.target.value })} /> </form> <br /> <div> Entered Value: {this.state.inputValue} </div> </div> ); } } ReactDOM.render(<App />, document.querySelector('#root')); |
Output:
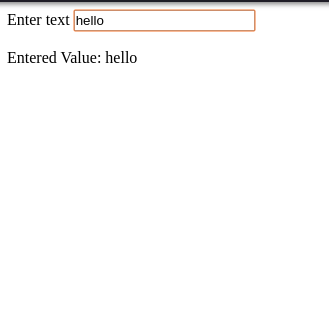
Example 3: Here we just added the onSubmit event handler which calls the function onFormSubmit and it prevents the browser from submitting the form and reloading the page and changing the input and output value to ‘Hello World!’.
src index.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; class App extends React.Component { state = { inputValue: '' }; onFormSubmit = (event) => { event.preventDefault(); this.setState({ inputValue: 'Hello World!' }); } render() { return ( <div> <form onSubmit={this.onFormSubmit}> <label> Enter text </label> <input type="text" value={this.state.inputValue} onChange={(e) => this.setState( { inputValue: e.target.value })} /> </form> <br /> <div> Entered Value: {this.state.inputValue} </div> </div> ); } } ReactDOM.render(<App />, document.querySelector('#root')); |
Output:
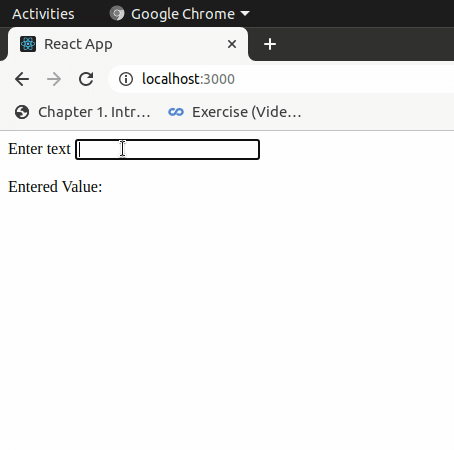