In this article, we will learn about ReactJS components, their types and how to render them.
More on React Components class, props, events
What is a ReactJS Component?
A Component is one of the core building blocks of React. In other words, we can say that every application you will develop in React will be made up of pieces called components. Components make the task of building UIs much easier. You can see a UI broken down into multiple individual pieces called components and work on them independently and merge them all in a parent component which will be your final UI.
You can see in the below image we have broken down the UI of GeeksforGeeks’s homepage into individual components.
.jpg)
Components in React basically return a piece of JSX code that tells what should be rendered on the screen.
Types of components in ReactJS
In React, we mainly have two types of components:
Functional Components: Functional components are simply javascript functions. We can create a functional component in React by writing a javascript function. These functions may or may not receive data as parameters, we will discuss this later in the tutorial. The below example shows a valid functional component in React:function demoComponent() {
return (<h1>
Welcome Message!
</h1>);
}
Class Components: The class components are a little more complex than the functional components. The functional components are not aware of the other components in your program whereas the class components can work with each other. We can pass data from one class component to another class component. We can use JavaScript ES6 classes to create class-based components in React. The below example shows a valid class-based component in React: class Democomponent extends React.Component {
render() {
return <h1>Welcome Message!</h1>;
}
}
The components we created in the above two examples are equivalent, and we also have stated the basic difference between a functional component and a class component. We will learn about more properties of class-based components in further tutorials.
For now, keep in mind that we will use functional components only when we are sure that our component does not require interacting or work with any other component. That is, these components do not require data from other components however we can compose multiple functional components under a single functional component.
We can also use class-based components for this purpose but it is not recommended as using class-based components without need will make your application in-efficient.
Rendering Components in ReactJS
React is also capable of rendering user-defined components. To render a component in React we can initialize an element with a user-defined component and pass this element as the first parameter to ReactDOM.render() or directly pass the component as the first argument to the ReactDOM.render() method.
The below syntax shows how to initialize a component to an element:
https://66f590c01003ab07b86e65a05079d55e.safeframe.googlesyndication.com/safeframe/1-0-40/html/container.htmlconst elementName = <ComponentName />;
In the above syntax, the ComponentName is the name of the user-defined component.
Note: The name of a component should always start with a capital letter. This is done to differentiate a component tag from HTML tags.
The below example renders a component named Welcome to the Screen:
Open your index.js file from your project directory, and make the given below changes:
src index.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; // This is a functional component const Welcome = () => { return <h1>Hello World!</h1> } ReactDOM.render( <Welcome />, document.getElementById("root") ); |
Output:
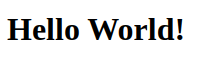
Let us see step-wise what is happening in the above example:
- We call the ReactDOM.render() as the first parameter.
- React then calls the component Welcome, which returns <h1>Hello World!</h1>; as the result.
- Then the ReactDOM efficiently updates the DOM to match with the returned element and renders that element to the DOM element with id as “root”.
ReactJS | Components – Set 2
In our previous article on ReactJS | Components we had to discuss components, types of components, and how to render components. In this article, we will see some more properties of components.
Composing Components: Remember in our previous article, our first example of GeeksforGeeks’s homepage which we used to explain components? Let’s recall what we have told, “we can merge all of these individual components to make a parent component”. This is what we call composing components. We will now create individual components named Navbar, Sidebar, ArticleList and merge them to create a parent component named App and then render this App component.
The below code in the index.js file explains how to do this:
Filename- App.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; // Navbar Component const Navbar=()=> { return <h1>This is Navbar.< /h1> } // Sidebar Component const Sidebar=()=> { return <h1>This is Sidebar.</h1> } // Article list Component const ArticleList=()=> { return <h1>This is Articles List.</h1> } // App Component const App=()=> { return( <div> <Navbar /> <Sidebar /> <ArticleList /> </div> ); } ReactDOM.render( <App />, document.getElementById("root") ); |
Output:
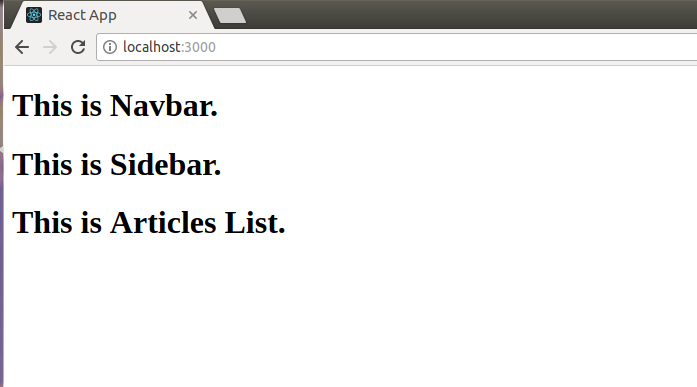
You can see in the above output that everything worked well, and we managed to merge all the components into a single component App.
Decomposing Components: Decomposing a Component means breaking down the component into smaller components. We have told the thing about composing smaller components to build a parent component from the very start when we started discussing components repeatedly. Let us see why there is a need to do so. Suppose we want to make a component for an HTML form. Let’s say our form will have two input fields and a submit button. We can create a form component as shown below:
Filename- App.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; const Form=()=> { return ( <div> <input type = "text" placeholder = "Enter Text.." /> <br /> <br /> <input type = "text" placeholder = "Enter Text.." /> <br /> <br /> <button type = "submit">Submit</button> </div> ); } ReactDOM.render( <Form />, document.getElementById("root") ); |
Output:
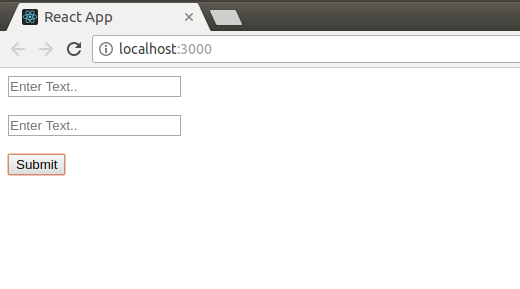
The above code works well to create a form. But let us say now we need some other form with three input fields. To do this we will have to again write the complete code with three input fields now. But what if we have broken down the Form component into two smaller components, one for the input field and another one for the button? This could have increased our code reusability to a great extent. That is why it is recommended to React to break down a component into the smallest possible units and then merge them to create a parent component to increase the code modularity and reusability. In the below code the component Form is broken down into smaller components Input and Button.
Filename- App.js:
- javascript
import React from 'react'; import ReactDOM from 'react-dom'; // Input field component const Input=()=> { return( <div> <input type="text" placeholder="Enter Text.." /> <br /> <br /> </div> ); } // Button Component const Button=()=> { return <button type = "submit">Submit</button>; } // Form component const Form=()=> { return ( <div> <Input /> <Input /> <Button /> </div> ); } ReactDOM.render( <Form />, document.getElementById("root") ); |
Output:
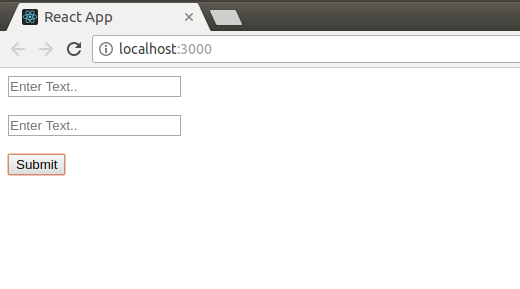
Till now, we have worked with Components with only static data. That is, we are writing data directly inside a Component. What if, we want to pass some data to our Components? React allows us to do so with the help of another property called props. We will learn about props in detail in our next article.
ReactJS Functional Components
In this article, we will learn about funcitonal components in React, different ways to call the functional component and also learn how to create the functional components. We will also demonstrate the use of hooks in functional components
Functional components in React
ReactJS Functional components are some of the more common components that will come across while working in React. These are simply JavaScript functions. We can create a functional component in React by writing a JavaScript function. These functions may or may not receive data as parameters. In the functional Components, the return value is the JSX code to render to the DOM tree.
Ways to call the functional component:
We can call the functions in javascript in other ways as follows:
1. Call the function by using the name of the function followed by the Parentheses.
// Example of Calling the function with function name followed by Parentheses
import React from ‘react’;
import ReactDOM from ‘react-dom/client’;
function Parentheses() {
return (<h1>
We can call function using name of the
function followed by Parentheses
</h1>);
}
const root = ReactDOM.createRoot(document.getElementById(‘root’));
root.render(Parentheses());
2. Call the function by using the functional component method.
// Example of Calling the function using component call
import React from ‘react’;
import ReactDOM from ‘react-dom/client’;
function Comp() {
return (<h1> As usual we can call the function using component call</h1>);
}
const root = ReactDOM.createRoot(document.getElementById(‘root’));
root.render(<Comp />);
Now, We will use the functional component method to create a program and see how functional components render the component in the browser.
Program to demonstrate the creation of functional components
- Open your React project directory and go to the src folder then edit the index.js file.
- Javascript
//index.js File import React from 'react'; import ReactDOM from 'react-dom'; import Demo from './App'; ReactDOM.render( <React.StrictMode> <Demo /> </React.StrictMode>, document.getElementById('root') ); |
- Open the App.js file from the src folder and edit the file.
- Javascript
//App.js File import React from 'react'; import ReactDOM from 'react-dom'; const Demo=()=>{return <h1>Welcome to GeeksforGeeks</h1>}; export default Demo; |
Output: After editing both files check the output in the browser. You will get the output as shown below.
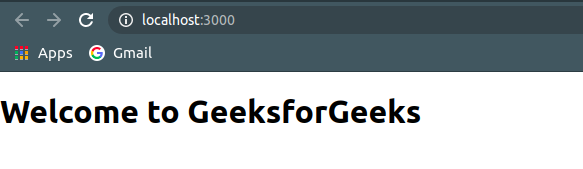
Problem with using functional components
Functional components lack a significant amount of features as compared to class-based components and they do not have access to dedicated state variables like class-based components.
Advantage of using hooks in functional components
The problem discussed above is solved with the help of a special ReactJS concept called “hooks”. ReactJS has access to a special hook called useState(). The useState() is used to initialize only one state variable to multiple state variables. The first value returned is the initial value of the state variable, while the second value returned is a reference to the function that updates it.
Program to demonstrate the use of useState() hook
Filepath- src/index.js: Open your React project directory and edit the Index.js file from the src folder.
- Javascript
//index.js File import React from 'react'; import ReactDOM from 'react-dom'; import Example from './App'; ReactDOM.render( <React.StrictMode> <Example /> </React.StrictMode>, document.getElementById('root') ); |
Filepath- src/App.js: Open your React project directory and edit the App.js file from the src folder:
- Javascript
//App.js File import React, { useState } from 'react'; const Example = () => { const [change, setChange] = useState(true); return ( <div> <button onClick={() => setChange(!change)}> Click Here! </button> {change ? <h1>Welcome to GeeksforGeeks</h1> : <h1>A Computer Science Portal for Geeks</h1>} </div> ); } export default Example; |
Output: You will get the output as shown below.
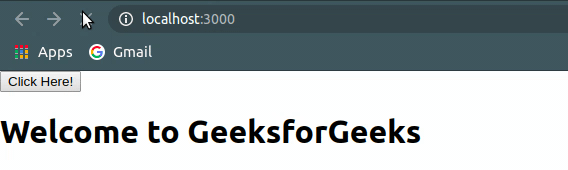
Functional components do not have access to lifecycle functions like class-based components do since lifecycle functions need to be defined within the boundaries of a class. A special React hook called useEffect() needs to be used. It is worth noting that useEffect() isn’t an exact duplicate of the lifecycle functions – it works and behaves in a slightly different manner.
Program to demonstrate the use of useEffect() hook
- Filepath- src/index.js: Open your React project directory and edit the index.js file from the src folder.
- Javascript
//index.js File import React from 'react'; import ReactDOM from 'react-dom'; import Example from './App'; ReactDOM.render( <React.StrictMode> <Example /> </React.StrictMode>, document.getElementById('root') ); |
- Filepath- src/App.js: Open your React project directory and edit the App.js file from the src folder.
- Javascript
//App.js File import React, { useEffect } from 'react'; const Example = () => { useEffect(() => { console.log("Mounting..."); }); return ( <h1> Geeks....! </h1> ); } export default Example; |
Output: you will get the output like this in your browser.
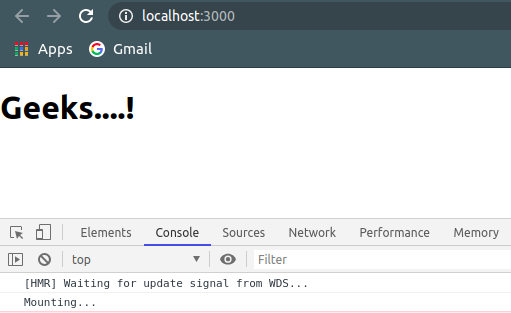
Data is passed from the parent component to the child components in the form of props. ReactJS does not allow a component to modify its own props as a rule. The only way to modify the props is to change the props being passed to the child component. This is generally done by passing a reference of a function in the parent component to the child component.
Program to demonstrate the use of props
- Filepath- src/index.js: Open your React project directory and edit the Index.js file from the src folder.
- Javascript
//index.js File import React from 'react'; import ReactDOM from 'react-dom'; import Example from './App'; ReactDOM.render( <React.StrictMode> <Example /> </React.StrictMode>, document.getElementById('root') ); |
- Filepath- src/App.js: Open your React project directory and edit the App.js file from the src folder.
- Javascript
import React, { useState } from 'react'; import props from 'prop-types'; const Example = () => { return ( <h1>{props.data}</h1> ); } function propsExample() { const [change, setChange] = useState(true); return ( <div> <button onClick={() => setChange(!change)}> Click Here! </button> {change ? <Example data="Welcome to GeeksforGeeks" /> : <Example data="A Computer Science Portal for Geeks" />} </div> ); } export default Example; |
Output: You will see the output like this in your browser.
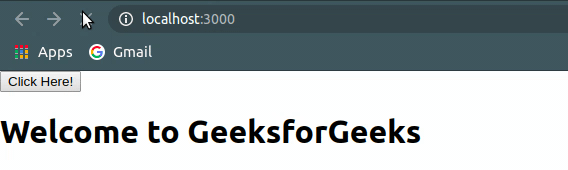